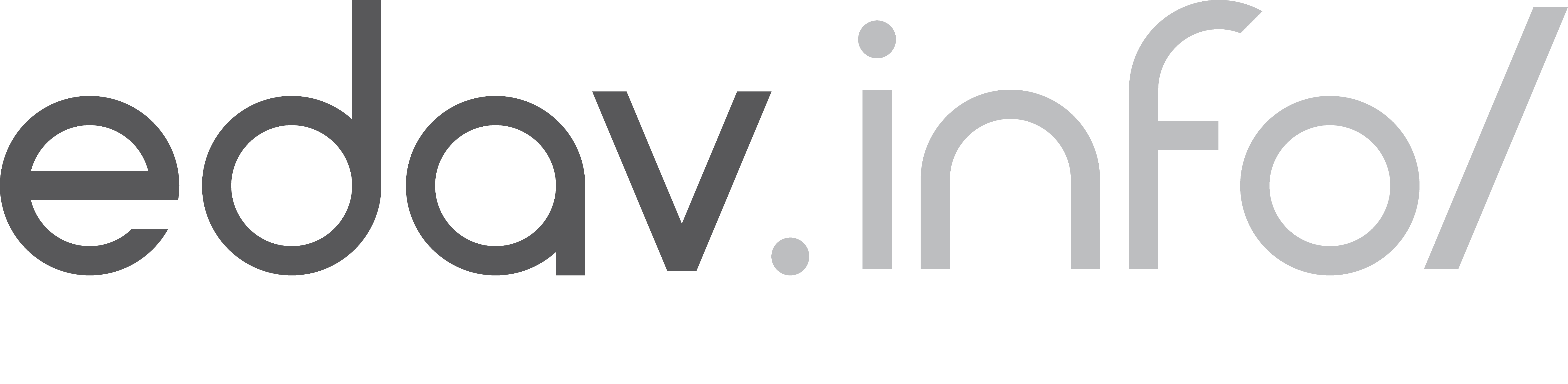
18 Interactive Networks
18.1 visNetwork (interactive)
visNetwork
is a powerful R implementation of the interactive JavaScript vis.js
library; it uses tidyverse
piping: VisNetwork Docs.
–> The Vignette has clear worked-out examples: https://cran.r-project.org/web/packages/visNetwork/vignettes/Introduction-to-visNetwork.html
The visNetwork
documentation doesn’t provide the same level of explanation as the original, so it’s worth checking out the vis.js
documentation as well:
http://visjs.org/index.html In particular, the interactive examples are particularly useful for trying out different options. For example, you can test out physics options with this network configurator.
18.1.1 Minimum working example
Create a node data frame with a minimum one of column (must be called id
) with node names:
# nodes
<- data.frame(id = c("The Bronx", "Manhattan", "Queens", "Brooklyn", "Staten Island")) boroughs
Create a separate data frame of edges with from
and to
columns.
# edges
<- data.frame(from = c("The Bronx", "The Bronx", "Queens", "Queens", "Manhattan", "Brooklyn"), to = c("Manhattan", "Queens", "Brooklyn", "Manhattan", "Brooklyn", "Staten Island")) connections
Draw the network with visNetwork(nodes, edges)
library(visNetwork)
visNetwork(boroughs, connections)
Add labels by adding a label column to nodes
:
library(dplyr)
<- boroughs %>% mutate(label = id)
boroughs visNetwork(boroughs, connections)
18.1.2 Performance
visNetwork
can be very slow.
%>% visPhysics(stabilization = FALSE)
starts rendering before the stabilization is complete, which does actually speed things up but allows you to see what’s happening, which makes a big difference in user experience. (It’s also fun to watch the network stabilize). Other performance tips are described here.
18.1.3 Helpful configuration tools
%>% visConfigure(enabled = TRUE)
is a useful tool for configuring options interactively. Upon completion, click “generate options” for the code to reproduce the settings. More here (Note that changing options and then viewing them requires a lot of vertical scrolling in the browser. I’m not sure if anything can be done about this. If you have a solution, let me know!)
18.1.4 Coloring nodes
Add a column of actual color names to the nodes data frame:
<- boroughs %>% mutate(is.island = c(FALSE, TRUE, FALSE, FALSE, TRUE)) %>% mutate(color = ifelse(is.island, "blue", "yellow"))
boroughs visNetwork(boroughs, connections)
18.1.5 Directed nodes (arrows)
visNetwork(boroughs, connections) %>%
visEdges(arrows = "to;from", color = "green")
18.1.6 Turn off the physics simulation
It’s much faster without the simulation. The nodes are randomly placed and can be moved around without affecting the rest of the network, at least in the case of small networks.
visNetwork(boroughs, connections) %>%
visEdges(physics = FALSE)
18.1.7 Grey out nodes far from selected (defined by “degree”)
(Click a node to see effect.)
# defaults to 1 degree
visNetwork(boroughs, connections) %>%
visOptions(highlightNearest = TRUE)
# set degree to 2
visNetwork(boroughs, connections) %>%
visOptions(highlightNearest = list(enabled = TRUE,
degree = 2))
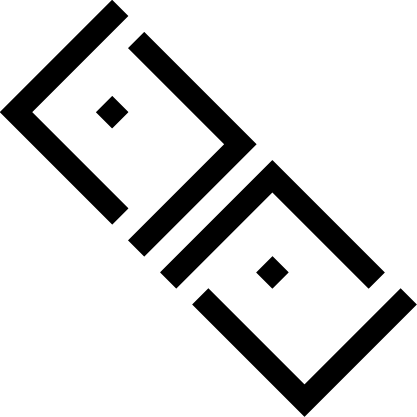
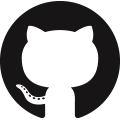
with